A Firebase programmatic UIKit chat app for people who have strange friends. Users can register their account, login, add friends via their email address, add their friends' profile image and chat. All data is synced to the Firebase Firestore database.
Here are some of the tools and techniques I used:
- UIKit - Programmatic UI (only one storyboard just for the launch screen)
- MVC design pattern
- Delegate/Protocol pattern
- Async/Await
- Firebase SDK (Firebase Auth & Firestore DB)
- Cocoapods
- Files Directory for saving and loading profile images
The app has several view controllers - welcome view controller, login/register, add/edit friends, list of friends and messages view controller. I did my best to separate the logic of the app from the views and user actions on view controllers. For the most part I used async/await as much as I could for the Firebase operations and sometimes escaping closures for certain functions like loading messages from the Firestore database.
Currently, this app does not support notifications and I might implement that in the future.
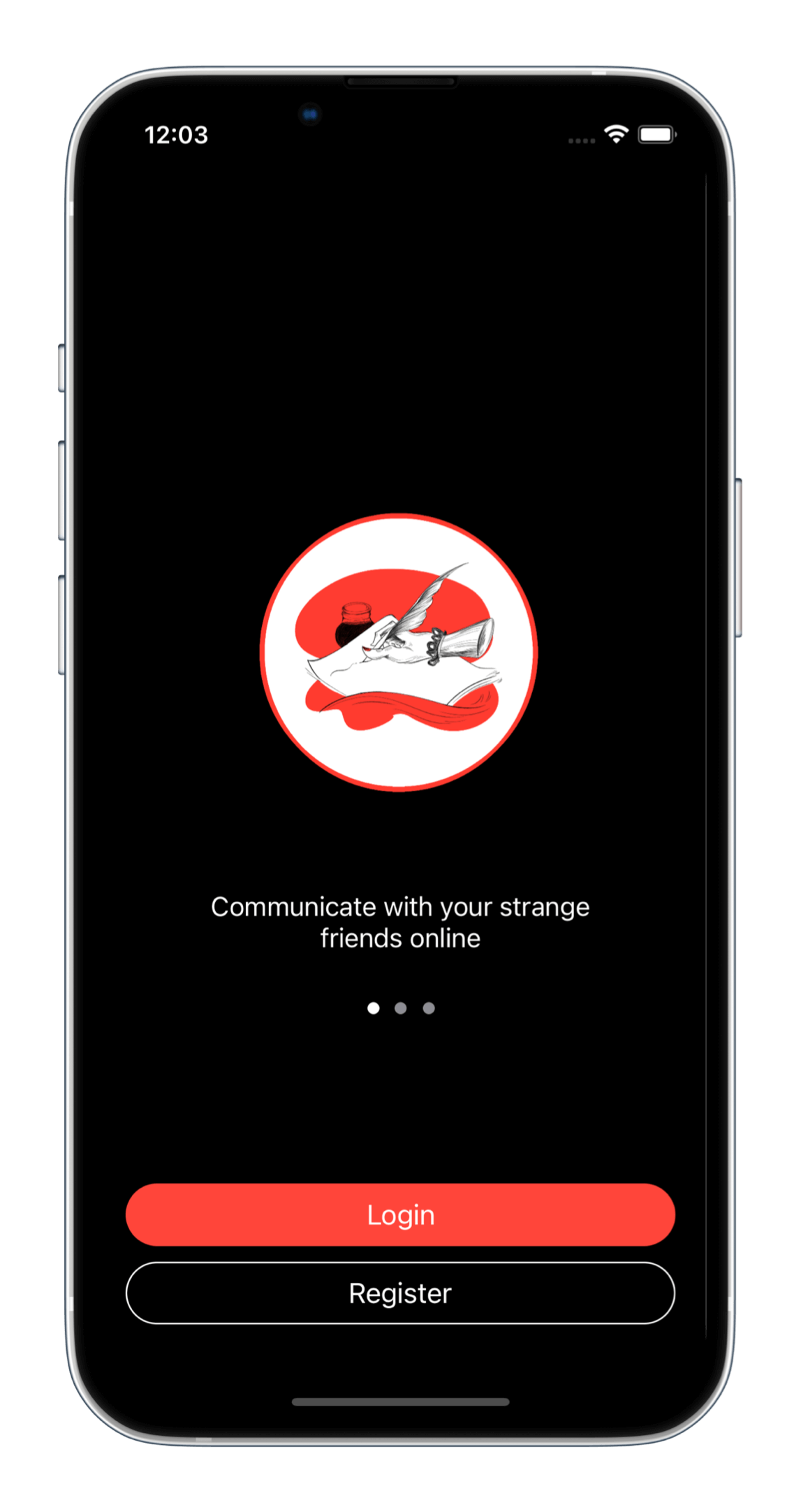
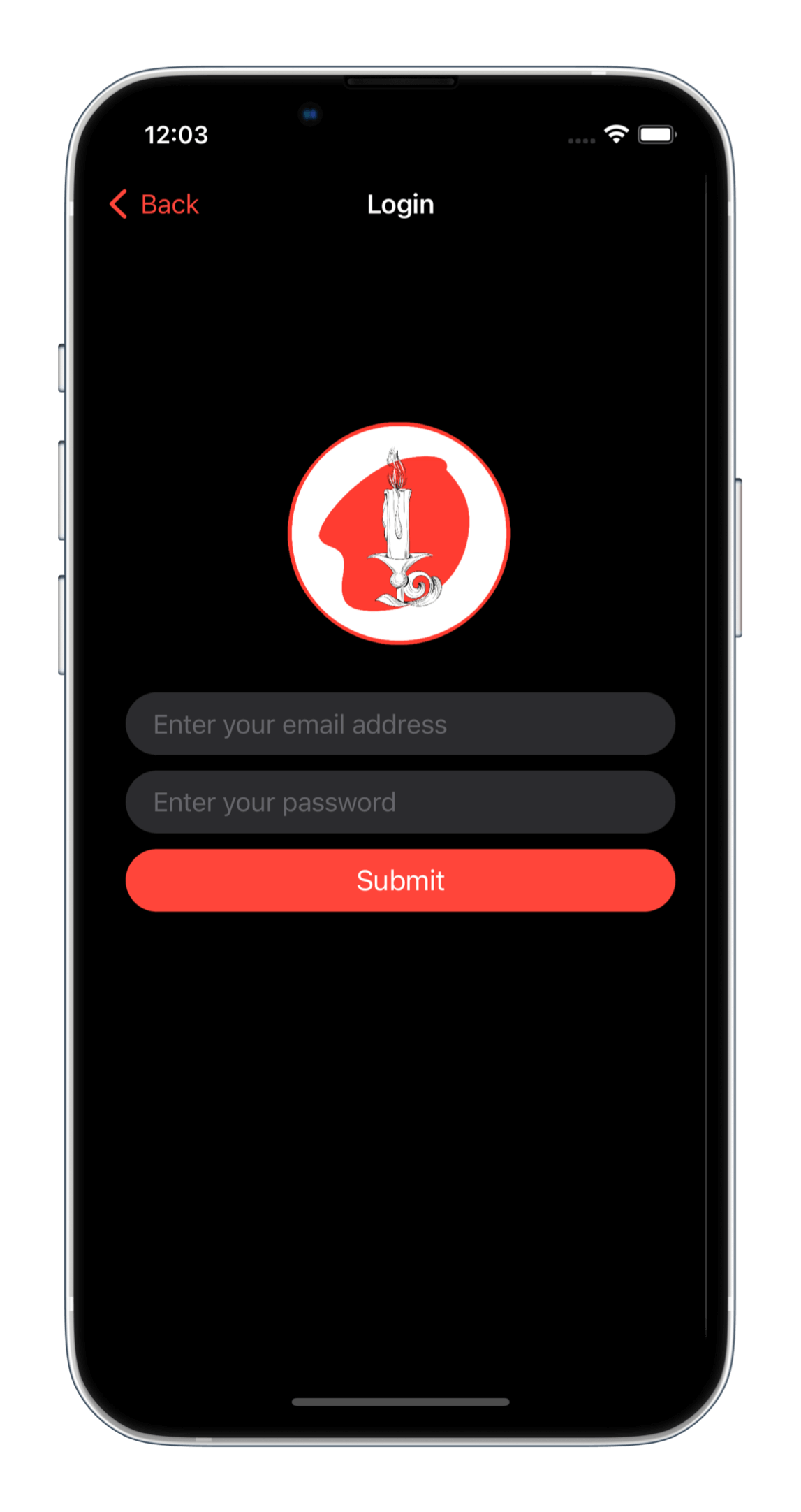
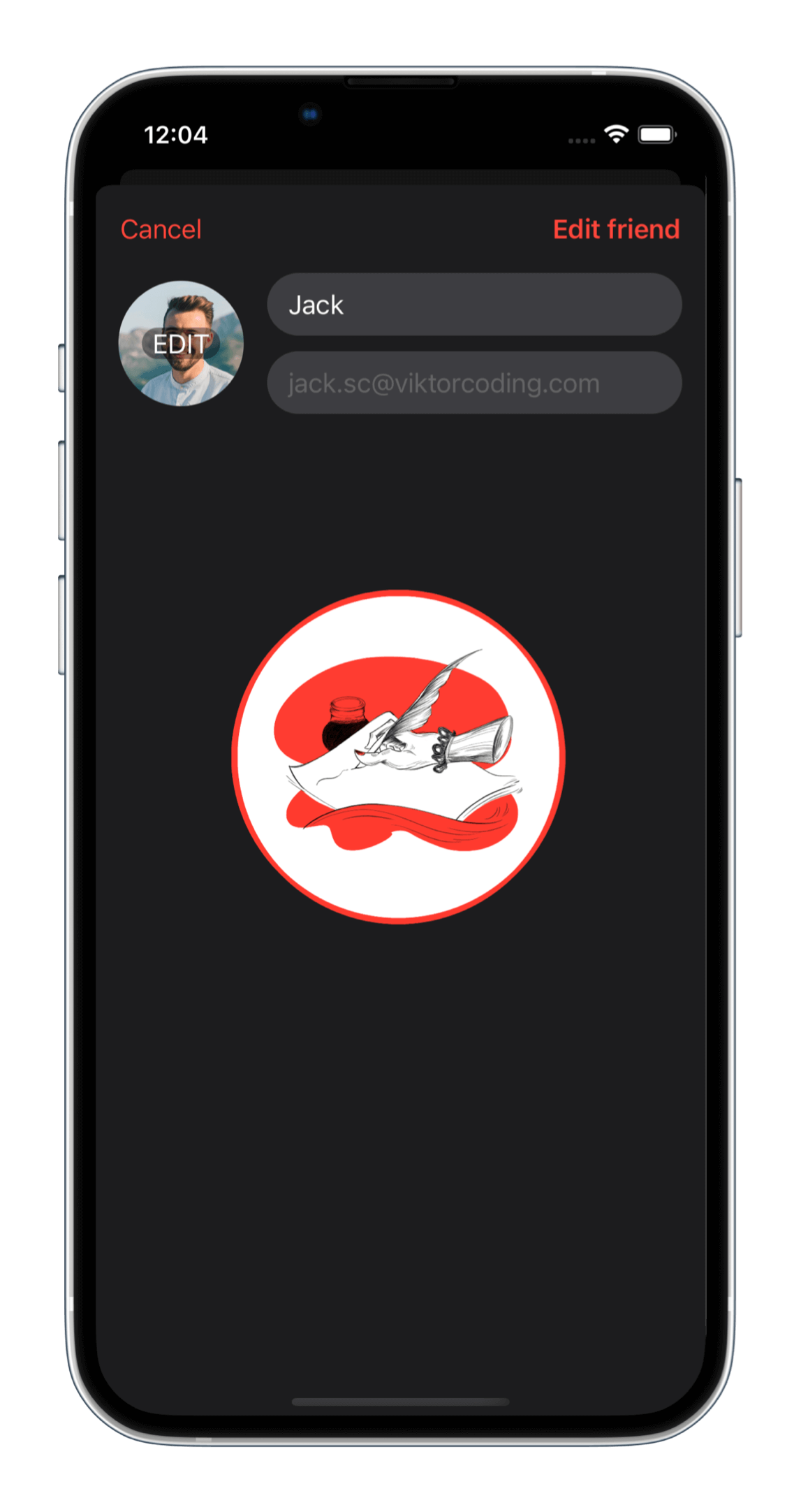
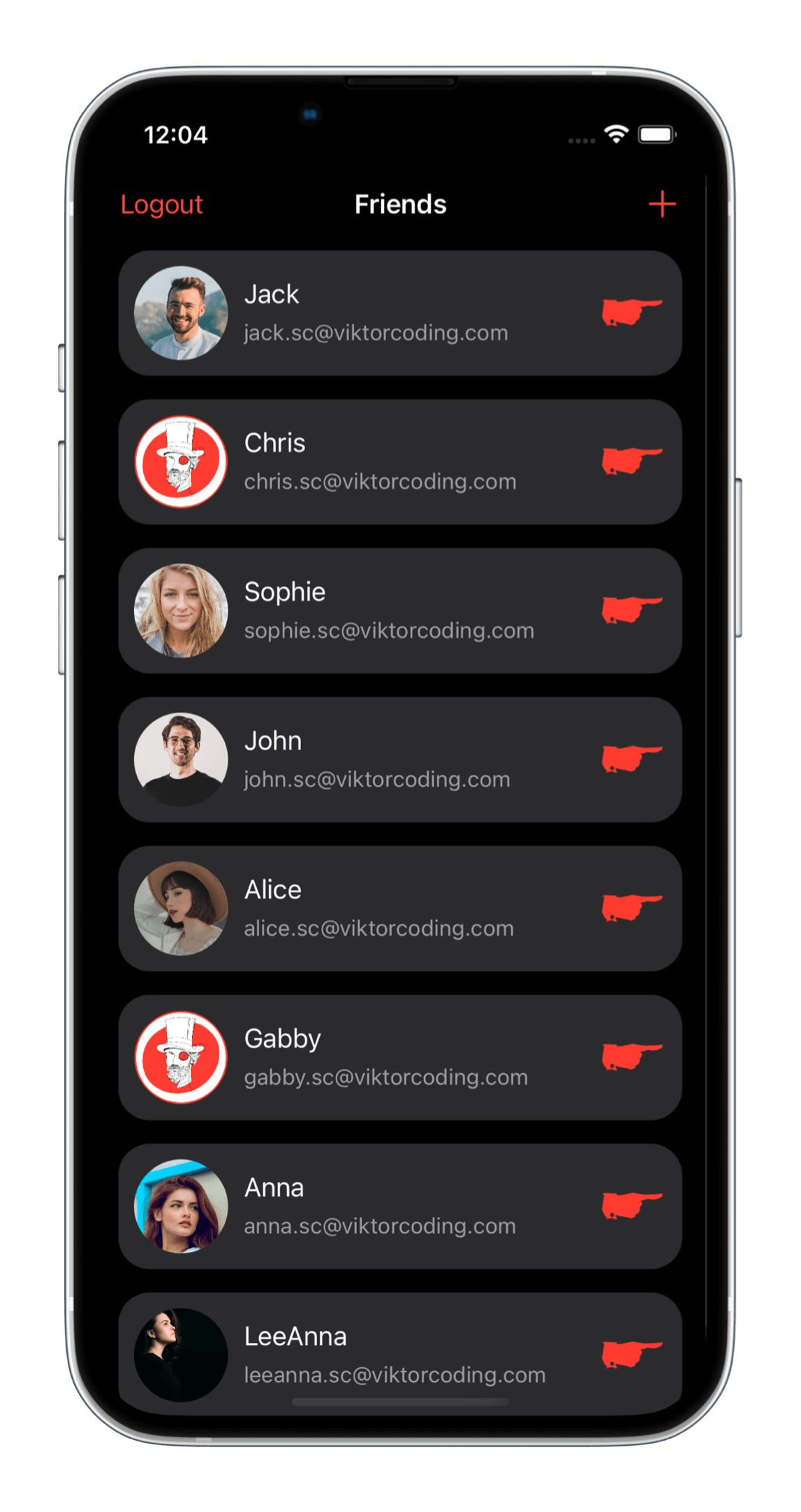
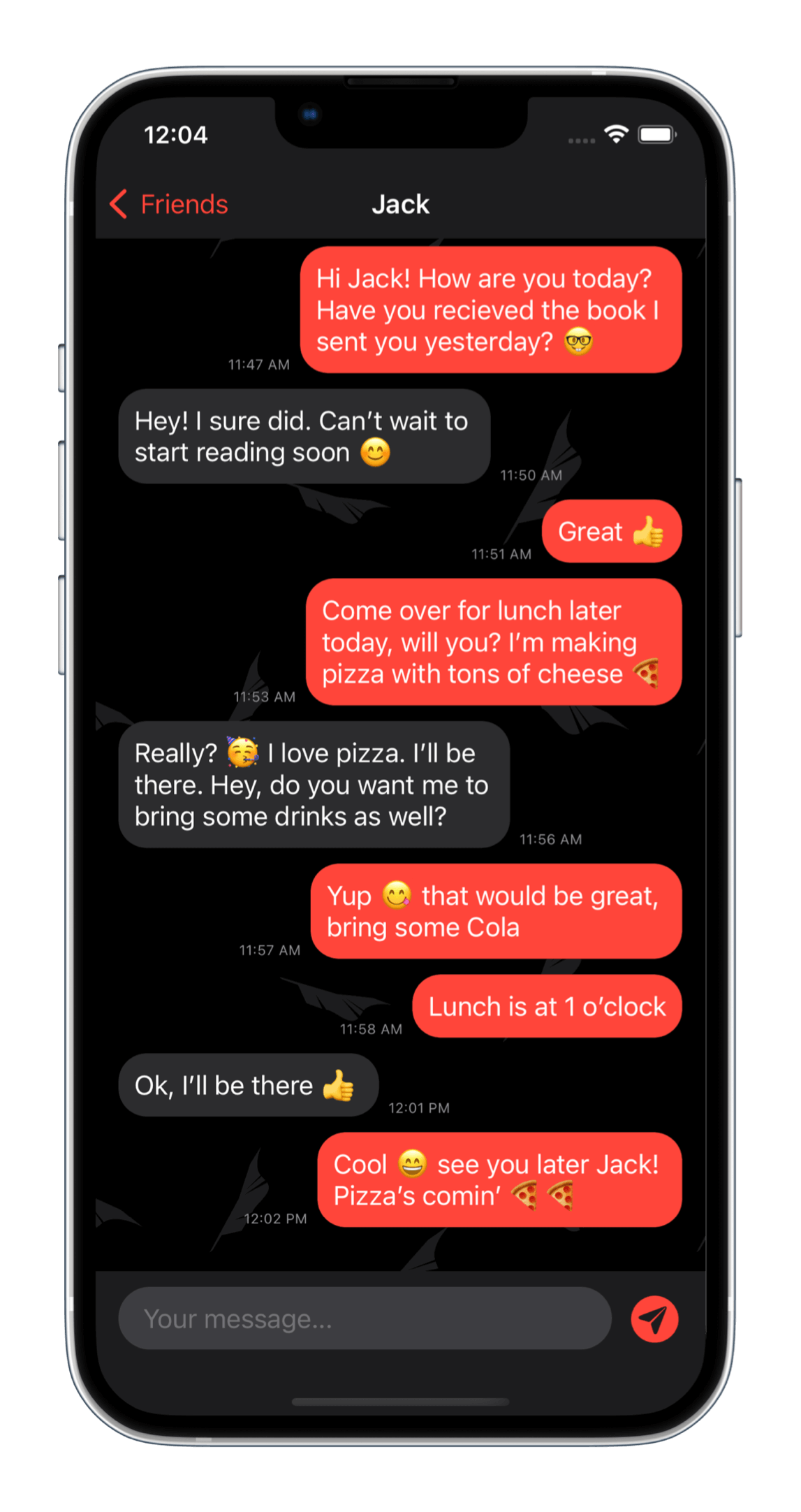
I did my best to set constraints programmatically so the app works on all iPhone screen sizes in portrait mode. I did the same for the custom cells for friends list and messages as well.
The app supports editing a friend (name & profile photo) and deleting already added friends by swipe actions. All changes are updated in the Firestore database in real time. The profile photo chosen by the user (directly from a camera or from the library) is saved in the files directory of the app on device. However, the unique identifier of the photo is saved in the Firestore database as a string reference so it can be loaded from the directory. Upon editing the profile photo, the old photo used for that particular friend is deleted from the directory and replaced with the new one unless the picture is the default one which comes from the assets catalog.
Every message has its own timestamp saved in the database. I used a computed property in the Message model to format the time each message is sent so it shows the time in the UI correctly.
The app performs a check each time a user adds a friend to see if the added user exists in the database at all (text field updates as the user types the email address) and if the friend being added already added the user as a friend before. Based on this check the app creates a new collection in the Firestore database where messages between the two will be saved. If the collection already exists, the added friend gets a reference to the exact collection as a string property. This way, there will be no duplication in the DB and loading messages is easier.
What I learned building this app
This is the first time I tried programmatic UI in UIKit so it took some research, but this is what I took from it all:
- How to separate concerns regarding the app logic (communicating with Firestore database) and visual representation of the data
- How to work with Firebase authentication, register users via email address and password, login and log out those users and edit their data.
- How to create documents and collections in the Firestore database, use custom models to save and merge properties in those documents, edit and delete specific properties etc.
- How to use files directory to save data on device, load it as its needed and delete it
- How to use swipe actions on cells in table view controllers
- How to create custom table view cells
- How to work with navigation controllers without using storyboards
- How to use Scene Delegate to setup a project for programmatic UI
- How to use weak self to prevent memory problems
- How to debug and check for memory problems in Xcode
I learned a lot building this app and I'm sure many things could be done better, but for the moment I am happy with how it turned out and that it works. 😅
In the future, I would love to add real time notifications as messages come in, build caching and more comprehensive error handling. At the moment, the app uses simple alerts to notify user about problems.
Special thanks to my sister, Ivana Maric of LePunktNoirStudio for creating all the illustrations for this app. We both love creepy stuff and that's why the app has its name and visual style. Check out her portfolio here.
Check out the project on my github repo: